Hello everyone! This is the 14th post in the node.js modules you should know about article series.
The first post was about dnode - the freestyle rpc library for node, the second was about optimist - the lightweight options parser for node, the third was about lazy - lazy lists for node, the fourth was about request - the swiss army knife of HTTP streaming, the fifth was about hashish - hash combinators library, the sixth was about read - easy reading from stdin, the seventh was about ntwitter - twitter api for node, the eighth was about socket.io that makes websockets and realtime possible in all browsers, the ninth was about redis - the best redis client API library for node, the tenth was on express - an insanely small and fast web framework for node, the eleventh was semver - a node module that takes care of versioning, the twelfth was cradle - a high-level, caching, CouchDB client for node, the thirteenth was jsonstream - streaming JSON parsing library.
Today I'm gonna introduce you to everyauth by Brian Noguchi. Everyauth is a connect middleware that allows you to setup authentication for your app via facebook, twitter, google, vimeo, tumblr, 4square, etc.
Here is a list of all the sites you can use to login into your app:
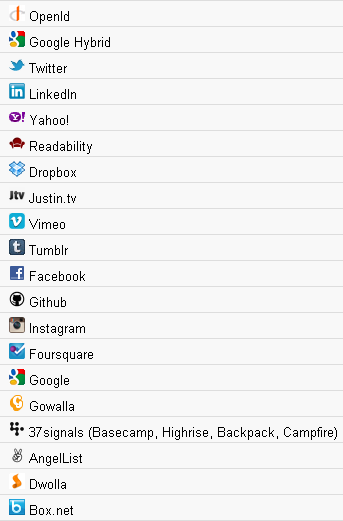
Everyauth supports OpenID, Google, Twitter, LinkedIn, Yahoo, Readability, Dropbox, Justin.tv, Vimeo, Tumblr, Facebook, Github, Instagram, Foursquare, Gowalla, 37signals, AngelList, Dwolla, Box.net.
Using it is as simple as setting up a middleware for connect:
var everyauth = require('everyauth');
var connect = require('connect');
var app = connect(everyauth.middleware());
And setting up config.json
that contains secret keys from the sites you are going to be using for authentication:
module.exports = {
fb: {
appId: '111565172259433'
, appSecret: '85f7e0a0cc804886180b887c1f04a3c1'
},
twit: {
consumerKey: 'JLCGyLzuOK1BjnKPKGyQ'
, consumerSecret: 'GNqKfPqtzOcsCtFbGTMqinoATHvBcy1nzCTimeA9M0'
},
github: {
appId: '11932f2b6d05d2a5fa18'
, appSecret: '2603d1bc663b74d6732500c1e9ad05b0f4013593'
},
// ...
};
Then you setup routes for logging in for each particular site you want to support (in this example facebook) and you're done:
var conf = require('./config.json');
var usersByFbId = {};
everyauth
.facebook
.appId(conf.fb.appId)
.appSecret(conf.fb.appSecret)
.findOrCreateUser(function (session, accessToken, accessTokenExtra, fbUserMetadata) {
return usersByFbId[fbUserMetadata.id] ||
(usersByFbId[fbUserMetadata.id] = addUser('facebook', fbUserMetadata));
})
.redirectPath('/');
You can install everyauth
through npm as always:
npm install everyauth
EveryAuth on GitHub: https://github.com/bnoguchi/everyauth.